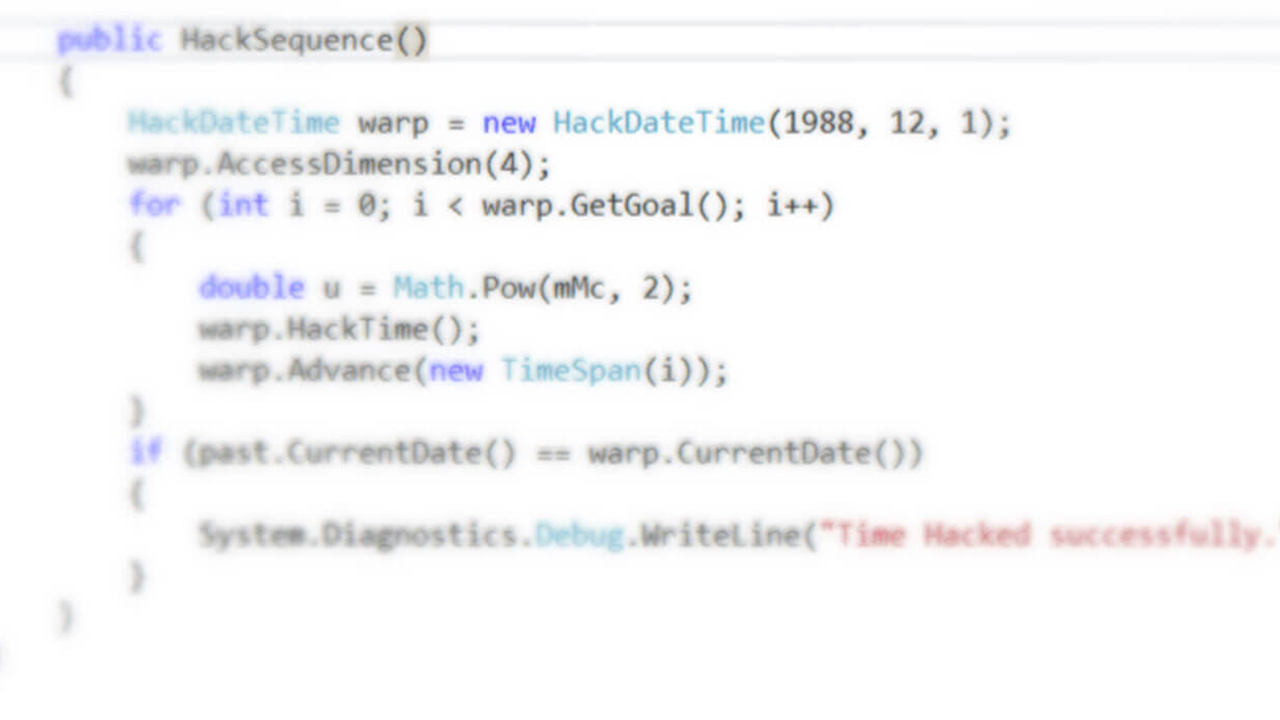
Mobile Development in .NET – Part 1 of 2147483648
I’ve been porting our Anyline SDK from Android/iOS to Xamarin and Windows Phone for the past months and it has been quite a journey so far. While still working on the Windows Phone release, I can definitely say that I’ve come a long way and I’m going to share my experience with you. So here’s the first blog post about my accomplishments, troubles, and anything in between in the world of .NET mobile development. This post, however, is dedicated to the basics, and I plan to write about more specific .NET-related topics in future posts.
So, What’s .NET?
Assuming that some of you have probably never worked with .NET or don’t exactly know what it is, I’ll give you a brief introduction.
.NET (pronounced “dotNET”) is a powerful framework developed by Microsoft and may even be considered an essential part of Microsoft Windows. It provides a controlled programming environment where software can be created and executed on Windows-based operating systems. For .NET development, the most substantial IDE is Microsoft Visual Studio. To give you a visualization, Figure 1 illustrates the main components of the .NET framework. The latest .NET version is 4.6.1 which has been released in November 2015, and you can look up the changes on Github, but we’ll stick to 4.5 for now.
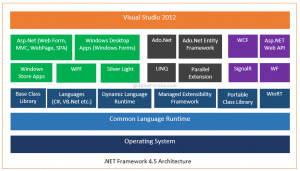
Software developed in .NET usually executes in the software-environment called CLR (Common Language Runtime), which is a virtual machine that provides memory management, garbage collection, thread management, type safety, exception-handling, labeling such code as “managed code”. Consider the CLR as the “engine” of the .NET Framework architecture.
Figure 2 visualizes how the CLR works:
- The managed code is compiled by the language compiler to so-called IL (Common Intermediate Language) code
- The IL code is then translated “Just-In-Time” to machine code.
This JIT compilation happens only the first time the code is called. Subsequently, calls to this code will execute the native code directly.
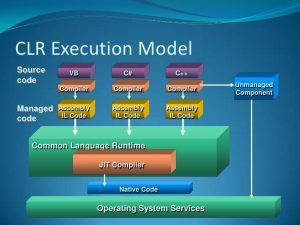
Another major component of the .NET framework is the so-called FCL (Framework Class Library), which is basically a vast set of classes, interfaces, and value types that provide access to system functionality. The BCL (Base Class Library) is a subset of the FCL and provides the most basic namespaces like System.IO or System.String, while the FCL provides a wider set of functionality like XML, ASP.NET, WinForms, and so on. You can look up the namespaces at MS as well.
So that’s a very brief overview of the most fundamental components of the .NET framework. I could probably write an in-depth blog post regarding .NET architecture in the future. For now, let’s not go too much into detail here and focus on the mobile aspect.
The Mobile Part
Mobile apps are usually categorized into three different types: Web apps, hybrid apps, and native apps. This distinction also applies to mobile .NET development. So, what options do we have when developing mobile .NET apps? It depends on what we want to accomplish. Generally speaking, we need to ask ourselves some important questions first:
Do I want to target multiple platforms (Android, iOS, Windows devices) at once, or maybe separately? Do I need lower-level functionality like device hardware access? What about performance, data storage, look and feel app distribution, and so forth? There is no such thing as a one-size-fits-all solution when it comes to mobile app development. Let’s have a look at the different types and see how they fit in .NET:
Web Apps
Mobile web apps are basically dynamic web pages with responsive UI, executed in the browser of a mobile device rather than being installed. If you want to develop a mobile web application, ASP.NET is the way to go. ASP.NET applications include web technologies like HTML5, CSS3, and JavaScript, and code-behind in C# or any other .NET programming language.
ASP.NET supports different development models: Web Pages, MVC (Model View Controller), and Web Forms. ASP.NET MVC can be distinguished in MVC3, MVC4, and MVC5 whereas from MVC4 on, additional features to support mobile apps are available.
- Pros
- Reuse of interface and core logic
- Much easier to maintain, as they have a common code base across multiple mobile platforms.
- Do not require store submission, thus no store approval
- Users don’t have to download web apps or update them on their devices
- Cons
- Limitations in terms of device APIs
- No store discoverability
- Limited to the capabilities of the mobile browser the user is using
Hybrid Apps
Hybrid apps are a mix of web and native apps. Similar to web apps, hybrid apps also use technologies like HTML, CSS, and JavaScript, but instead of targeting a mobile browser directly, they are wrapped inside a WebView which is hosted in a native container, giving them some device capabilities against “pure” web apps. This container may be Apache Cordova for example. Hybrid apps are a good choice if you want to provide a “simpler” app (no heavy device functionality) that is more of an informational nature but still can be used offline in comparison to a web app.
If you choose to develop a hybrid app in Visual Studio, you can check out Visual Studio Tools for Apache Cordova or Teleriks AppBuilder Extension.
- Pros
- Usually easier to develop than a native app
- Reusable code-base
- It may look and feel like a native app
- Lower budget costs from an organizational view
- Offline & Online
- Cons
- Still doesn’t look and feel as smooth as a truly native app
- Unique capabilities of different platforms require different plugins / platform-specific code
- Slower performance compared to native apps
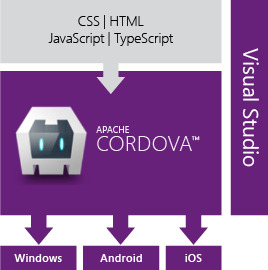
Native Apps
Generally speaking, native apps are built for devices of a particular platform, using specific SDKs, and have the ability to address all the device-specific hardware and software APIs. This gives them the power to offer the fastest, most reliable, and most responsive experience to users, compared to hybrid and web apps.
- Pros
- Fastest performance
- Most natural look and feel
- Full app store support & ability to provide updates
- Access to built-in device features
- Cons
- Requires more experienced developers
- higher costs / higher maintenance, especially for multiple target platforms
- Harder to create consistency across multiple platforms
Mobile apps in .NET are usually associated with the Windows (Phone) device family, but you should be very well aware that you can create native mobile .NET apps, written in C# for Android and iOS as well, thanks to Xamarin. You should be aware that if you decide to create an iOS app on a PC using Visual Studio and Xamarin, you’ll still need a Mac as a build host. Also, Xamarin is fairly expensive. Talking about Xamarin deserves an own blog post, though. Let’s stick to Windows for now.
I’m going to address Windows Phone 8.1 and Windows 10 Mobile, because hardly any device uses Windows Phone 8.0 or lower nowadays, and as of 21 January 2015, the Windows Phone brand is being phased out, making way for Windows 10. With Windows Phone 8.1, WinRT (Windows Runtime) is introduced to the mobile world of Windows, providing app development in C#, VB.NET, and the development of Windows Runtime components in C++/CX. With Windows 10, the UWP (Universal Windows Platform) is introduced as an extension/successor of WinRT.
As Figure 4 illustrates, most Windows-based mobile devices still run on Windows Phone 8.1, there are only a handful of devices (mostly of the Lumia family) that are Windows 10 Mobile compliant since it has only been released in Q4 2015.
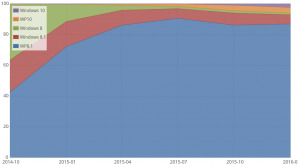
Microsoft made the terminology around “Apps” quite confusing, mainly because of their effort to converge mobile and desktop apps and because of the changes that Windows 8 brought. The term “Windows Store App” includes different kinds of apps:
- Windows Apps
- Windows 8.1 Applications that are based on WinRT and embrace the UI design style called “Metro Style” compared to traditional desktop applications.
- Windows Phone Apps
- Apps that are either based on WinRT (Windows Phone 8.1) or Silverlight (WP 7.x, WP 8.0)
- Universal Apps
- For Windows 8.1 / Windows Phone 8.1: Apps that are based on WinRT and run on both mobile and desktop by using a shared codebase
- For Windows 10: Apps that are based on the Universal Windows Platform (UWP) and run on both Windows 10 and Windows 10 Mobile
Microsoft makes it really hard to make sense of all its acronyms and names. For example, Windows RT and WinRT are two different things, but both mean “Windows Runtime”. Windows RT is an operating system optimized for mobile OEM Microsoft devices, whereas WinRT is the homogeneous application architecture that I briefly described earlier. When speaking of app distribution, the definition “Store” is also confusing thanks to Microsoft’s rebranding: “Windows Store” is the app store for primarily distributing metro-style apps for Windows 8.x, Windows Server 2012, Windows 10, and Windows 10 Mobile. “Windows Phone Store” is the mobile counterpart that offers Windows Phone 7.x and Windows Phone 8.x apps and is being discontinued/migrated in favor of the Windows Store.
Conclusion
Wow, this blog post turned out to be longer than it should, and we barely even scratched the surface. I hope I didn’t leave you too puzzled with that last paragraph because it can get quite confusing when it comes to the mobile world of Windows. Thank you for reading.