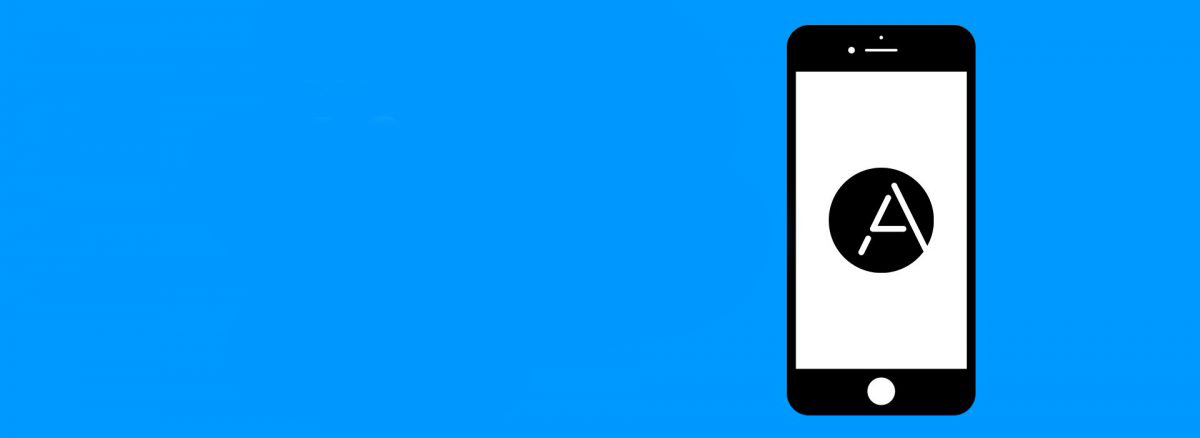
How to Build a Custom Use Case with the Anyline OCR SDK
Discover how to build your own custom use case fore mobile data capture with the Anyline mobile scanning SDK.
Hello and welcome to our third Anyline tutorial! We will show you how you can build a Custom Use Case with the Anyline OCR SDK! We are going to build a password scanner for preset wifi passwords. This is just one of the endless use cases you can build with the Anyline OCR module. Feel free to try it out, and show your creativity with your own scanning use case.
What we will need
Before we can start, you’ll need a couple of things like Android Studio, an Android device, a USB cable, and our Anyline Examples project. If you haven’t opened our Examples Project with Android Studio yet, please do so now. If you want some help with that, you can check out our previous video, which will guide you through all the steps.
Once you have finished this step and the Example Project is open in Android Studio we are ready to start.
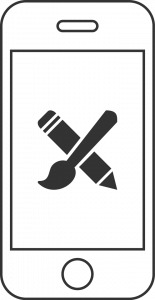
Getting started and adapting the view configuration
As already mentioned earlier, we’re going to build a Password Scanner Demo using the Anyline OCR Module. So first thing we’re going to do is to navigate to a similar example in the OCR package of the project. For this use case the best starting point is probably the ScanIbanActivity, so we’re just going to copy this ScanIbanActivity and create a new one, let’s call it “ScanWifiPasswordActivity”. We will just leave the standard layout and only adapt the ViewConfig to customize our ScanView.
The view config is set here. As you can see we set the config via json file, you could also do this using xml attributes, but we’re going to use the json file as well. You can find all view config files in the assets directory of your app. So let’s copy the iban_view_config and name it wifi_password_view_config.
If you have tried out our IBAN scanner in the examples app, you may have noticed that the cutout is very wide but not that high. For the password scanner we will probably need a higher cutout with about the same length. So we’re just going to change the ratioFromSize from 10:1 to let’s say 10:2. Also, we want to have sharper edges for the cutout corners, so we’ll use a cornerRadius of 3 instead.
Maybe let’s try out a different color for the cutout as well. We’re going use a light blue for the default color and green for the cutout feedback.
If you’re not happy with the other feedback options, you can change that as well. So let’s turn off the beep sound by setting this to false and maybe use a different animation, I personally like the resize animation, so let’s add the animation here and use a longer duration than the default of 75ms. We’ll simply set it to 150ms.
We do not need the fill color with this feedback style, that’s only necessary if you use contour_rect as animation style here, since we are using contour_points, we’re just going to delete the fillColor property, but you can also leave here, it will just be ignored.
Ok, I’m quite happy with this config, but if you want to make some more changes you can check out the complete list of available parameters in the Anyline View Configuration section of our Documentation.
Configuring the OCR Module
So we are already finished with the first half of our example, the next step is to configure the OCR module itself. For this, we have to change back to our ScanWifiPasswordActivity and find the anylineOcrConfig in the code.
As you can see here the ScanMode is set to LINE. This is perfect if you want to scan text with an unknown length. Since every password is different lengths, this setting is perfect for us.
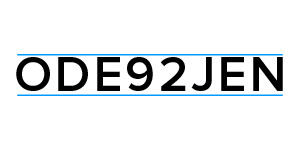
The other scanMode would be GRID. The grid mode is the right choice if you want to scan things with a constant layout like the bottlecap codes for example, where we always have a 3 by 3 grid.
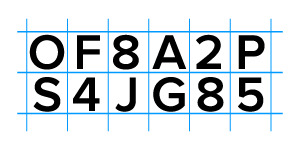
Next, we have to set the tesseract languages, we’re going to try it with the ones that are already defined here.
If you have a use case where those languages do not really work that well, we have a great tool to improve this. All you need is the font (or a similar font) that you want to scan and our trainyourtesseract.com site.
We’re not going to train a font, but let’s check out the site for a second. All you need to do is enter your email address, select what characters you want in your traineddata file, maybe you only need upper case letters, so you can deselect all the others.
Then you have to upload your font file and click on train now. You will get the traineddata file per mail within moments.
If you want to use your own font you have to copy this traineddata file in the assets/tessdata folder here. And then call the copyTrainedData function with your file and the md5 hash of this file as a string.
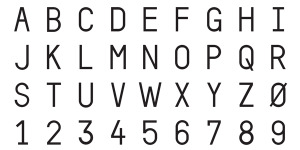
Next, we need to set our characterWhitelist. Since we hope for a secure password including upper and lower case character, numbers and all kinds of special characters, we either extend our character whitelist or we delete this setting completely, to allow all characters.
Note, that if you know the allowed characters, you should always restrict them with this parameter, because it improves the scan process significantly. But, since I don’t know what characters will be contained in the passwords, I am just going to delete it.
It is also important to limit the size of the characters we want to scan. If the min height is too low you might get a lot of small artifacts that can slow down the scan process. If it is too high it may filter out small characters and you may get wrong results or no result at all if you filter the characters out all together. Similar things apply for the max character height. So to find a good setting for those values it may take some trial and error attempts.
For the password scanner I will use a minCharacterHeight of 30 and leave the maxCharacterHeight at 60 for now. The confidence parameter gives a level of how likely the result will be correct. So if you set a value that is low, you may get a result quite fast, but it may not always be correct. If you set it very high, it will be more likely to get correct results but the scan process will take longer. I will just leave it at 65, which for me seems like a good compromise between accuracy and speed.
With the validation regex you can verify your result. Since we are looking for a random password we cannot use this. I am just going to delete it.
The “remove small contours” option is quite handy if you are looking for capital letters or numbers only. But if you deal with small letters or special characters you should probably set these options to false, because it can remove things like the dot on the small letter i, which can lead to wrong scan results.
Since we want to scan all kinds of characters I am going to set this to false. We will set the removeWhitespaces option to false as well, since passwords may contain whitespaces. And the last parameter is the minSharpness. This is to avoid the processing of blurry images. Since those images most probably will not lead to a valid scan result we want to skip them and only use relatively sharp images to speed up the scanning process. Again, you should go for a good compromise between sharpness and speed. So let’s leave this at 66. That’s it, we are done with the Anyline configuration part.
Run the password scanner on the device
Now all that’s left to do is add our Activity to our list view and we can try out the password scanner on the device. Let’s head to the example_activities.xml and create a new item in the example activity string array and call it “scan_wifi_passwords”.
The string will appear red and we’ll fix that in a second, but first let’s stay in that xml file and scroll down to the other string array for the activity classes and add our ScanWifiPasswordActivity there.
Now we’re finished here and we’ll add that scan_wifi_passwords string to the strings resource. The item in the ListView shall say “Scan Wifi Passwords”, and that’s what we’re going to type in there.
The activity will have a header label, which we will call “Wifi Passwords”. We’ll also add this in the strings.xml and call it title_wifi. Now the last thing we need to do is to navigate to the AndroidManifest.xml and actually add the activity there. That’s it. Let’s build the project and deploy it on our android device!
Try out scanning!
So here we are. The example app is up and running and we’re ready to try out the password scanner. What I’ve got here is the information on the bottom of a router, and I’ll just scan the network key from the label. Let’s place the password in the cutout and here we go. Works great – no typing necessary!
If you’ve read this and you’re curious about the Anyline SDK, go ahead: download it and try it out for free! And most important: if you like, give us feedback on the process!