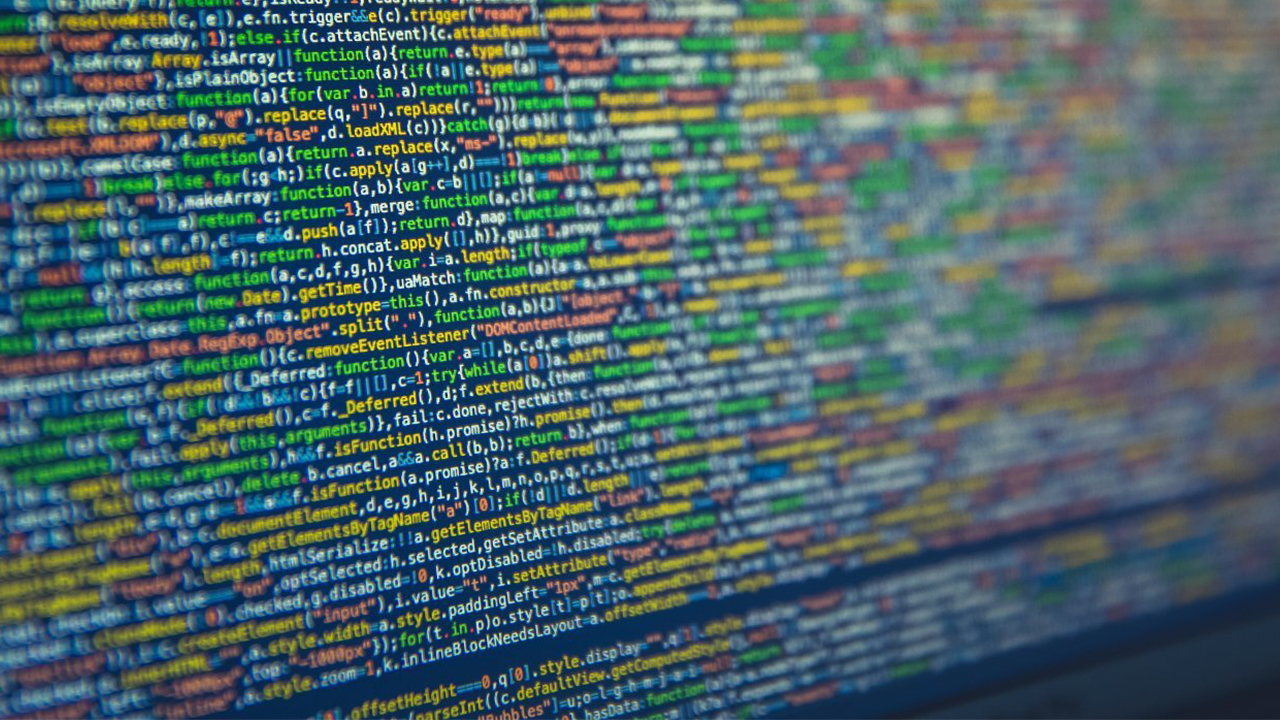
Develop React-Native Apps with Native Components in Javascript
Have you ever tried to develop a React-Native app or even combine it with native components? Find out how to get started.
After some struggling months of discovering the React world and also the native landscape, I’ve decided to write a blog post about my experience with implementing Anyline into React-Native. Many of you may have heard of React-Native or tried it out and already know the strengths and the weaknesses with it.
What is React?
React itself is just a View layer released by Facebook in 2013. Basically, it’s all about creating custom reusable DOM elements and combine them to a structure of data flow through your application. This is great because you can split up a big and complex WebApplication into smaller components. Each of those has its own environment called State. Also, you don’t have to worry about affecting other components by changing one component.
React comes with his custom Syntax JSX, which, in my opinion, is really awesome. It’s like combining HTML and Javascript in one Syntax. Every Component has a render function, which, depending on the state of the component (and so the information the component has), will render that particular state of the component, and also after updating the state elsewhere, it will show the current state.
This will lead you to the conclusion, that React isn’t rendering every component after every state update all the time, and that’s right. React uses a virtual DOM (Document Object Model) to prevent constant re-rendering. It finds the absolute minimal set of DOM mutations needed to display the next state and applies them. No manual DOM manipulation, no ugly two-way-data-binding, just updating single components, when they need to. Pretty cool, huh?
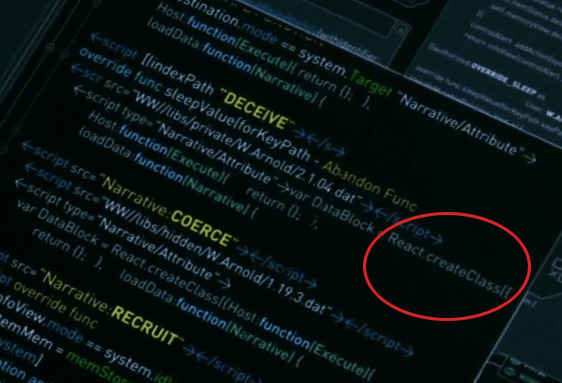
Also, if you have seen Westworld, every Host is built using React.
Wow, This React Has No Cons, Right?
Wrong! React is great, no question, but it also has its downsides. At first, learning React will take some time and will slow down your workflow tremendously in the beginning. Also, you will have to reinvent some wheels. The initial implementation and creating a good codebase to get started can be very time-consuming.
Anyway, you have to get structure into your app and define an architecture for managing your data flow (like Redux).
What is React-Native?
React-Native is like the conductor of native components. You write your Opera in Javascript and it will conduct the native components when, where, and how you want it. A bit more in detail, React-Native communicates over the React-Native Bridge with its own Javascript thread to all the native components in a native thread. It’s like a browser, but instead of rendering divs, tables, spans, or h3’s, it renders native components.
So, when you’re building your production-ready app, you will end up with the React-Native libraries and all your native components which are all compiled with your platform’s standard build tools. Additionally, you will get a big Javascript bundle that contains all your Javascript Code. When the application starts, React-Native will start the Javascript code that will define which components are to be created on the screen.
An interesting fact about this is that in debug mode, all your Javascript code runs on your main machine and is only provided through the wire. So you can use HotReloading without even restarting your app. Also during debug mode, you can run multiple devices even on different platforms (iOS, Android, or Web), which all react live on your Code Changes *Mind Blown*.
Ok, Enough “Each One Teach One”, How Did You Implement Anyline?
In React-Native you can implement Native Modules for Android and iOS. So you build your React-Native app and communicate with your native code over the React-Native Bridge Module. You now should just call native functions from Javascript, hand over parameters, and provide callbacks. With React-Native Link, every native dependency gets linked to your project. Easy, isn’t it!
With this, you can simply rebuild your app and refactor everything to Javascript piece by piece. This is also the case for complex native high-performance code that you don’t want to give out into the Javascript world. To give you some example code, here is a snippet from our React-Native Anyline Wrapper. We call a function to start the scan, that we want to pass through to our native component:
AnylineOCR.setupScanViewWithConfigJson(
JSON.stringify(config),
type,
this.onResult,
this.onError
);
in Android, we can get this call by:
@ReactMethod
public void setupScanViewWithConfigJson(String config, String scanMode, Callback onResultReact, Callback onErrorReact) {
...
}
and in iOS:
RCT_EXPORT_METHOD(setupScanViewWithConfigJson:(NSString*)config scanMode:(NSString *)scanMode
onResultCallback:(RCTResponseSenderBlock)onResult onErrorCallback:(RCTResponseSenderBlock)onError) {
...
}
So, Should I Use React-Native from Now On?
As always, it depends on your project and personal preferences. We’re quite deep into the React world and do think, that having a common codebase and split everything up into smaller components, is great for future feature implementation and of course the team development process.
You don’t have to renounce native functions as well and still have most of the native advantages. At first, you will have a rough start, but when you get into it, you don’t want to miss it!